Javascript Blackjack Dealer
Looking for a new project in JavaScript that I have not done in Python, I got to thinking about arrays.
Very simple JavaScript which simulates a game of Blackjack. JAVASCRIPT: Need help to writing a basic simulation of how a BlackJack dealer draws cards. Write a basic simulation of how a BlackJack dealer draws cards. Note: there is no user interaction here (no prompts or alerts, unless you're doing the Challenge portion), just output on the web page. I am currently learning JavaScript and the project I have been working on is creating a basic Blackjack game. Some code was provided for me, with the additional instructions: 'How would you edit the above code to PRINT what you and the Dealer have? How would you edit the code to let you know if you beat the dealer. Blackjack basics for players and dealers. The basic rules of blackjack are simple. Players try to score as close to 21 as possible without exceeding 21. In contrast to poker, where players compete with each other, blackjack is a one-on-one game between each player and the dealer. As the dealer, the basic rules of blackjack still apply. Free Blackjack Games 2020 - Play blackjack FREE with our instant, no registration games. Enjoy 60+ of the best blackjack games (choose from many variants).
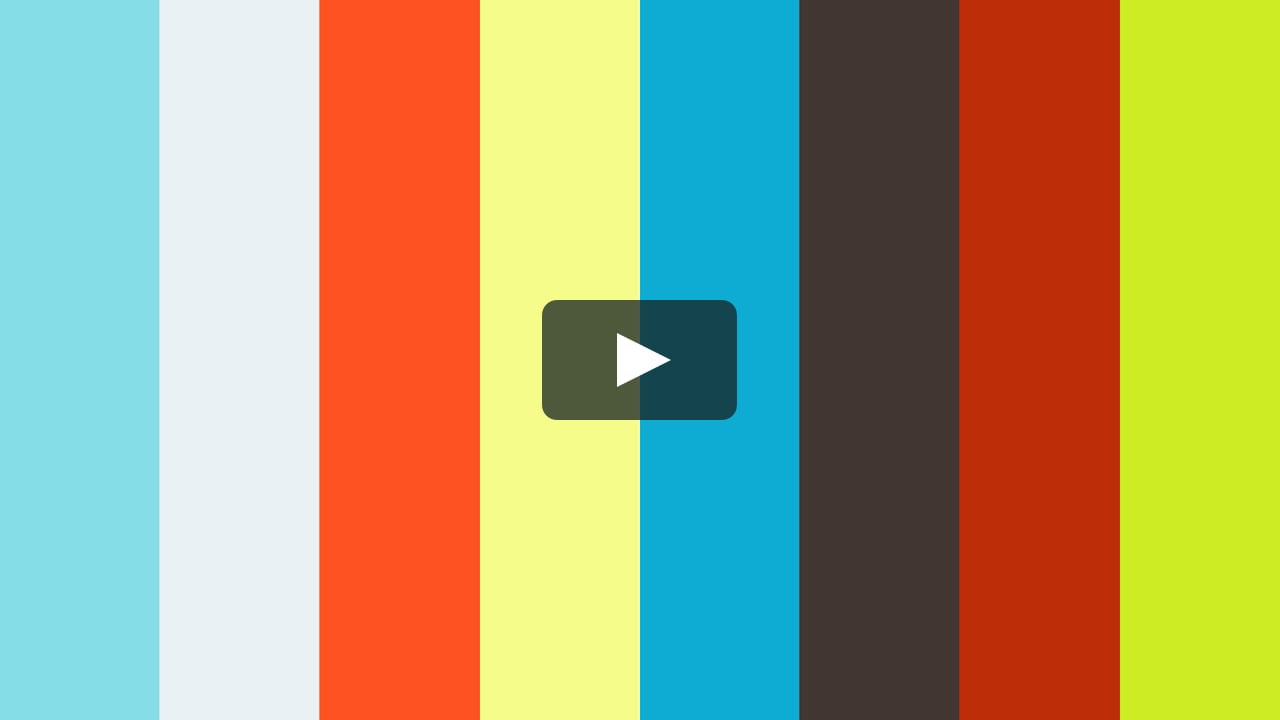
As JavaScript allows us to generate random numbers and store variables (see “Scissor, Paper, Stone”) it should allow me to also recreate the card game of Blackjack or 21. For more information on Blackjack please see: https://en.wikipedia.org/wiki/Blackjack
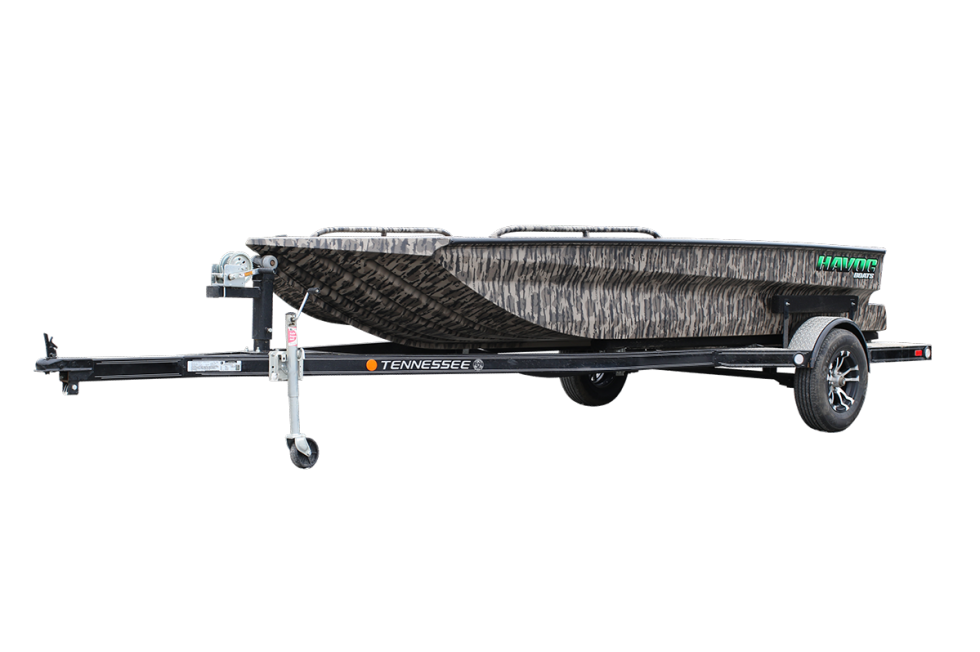
The smallest game of Blackjack requires 2 people – a player and a dealer.
The objective of Blackjack is for the player to either score 21 with 2 cards, or get a higher score than the dealer without going over 21. If the player goes over 21 then they lose. If the dealer goes over 21 then the banker loses.
The dealer is going to be controlled by the computer.
I’m going to use one deck of cards (52 cards). The 52 cards are split into 4 suites (Hearts, Diamonds, Spades, Clubs) with each suite containing 13 cards:
Ace, 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack, Queen, King
For this version of Blackjack I’m going to define an Ace as having the value of 1, and the Jack, Queen, King as having the value of 11.
So each suite now has;
Javascript Blackjack Dealer Log
1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 11, 11, 11
The values can be stored in an array, which we can call “deck”:
// variable to hold the deck of 52 cards
var deck = [1, 1, 1, 1, 2, 2, 2, 2, 3, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 6, 6, 6, 6, 7, 7, 7, 7, 8, 8, 8, 8, 9, 9, 9, 9, 10, 10, 10, 10, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11];
We then need JavaScript to randomly choose a card and remove the card from the possible cards that can be played.
// geektechstuff
// BlackJack
// variable to hold the deck of 52 cards
var deck = [1, 1, 1, 1, 2, 2, 2, 2, 3, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 6, 6, 6, 6, 7, 7, 7, 7, 8, 8, 8, 8, 9, 9, 9, 9, 10, 10, 10, 10, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11];
// variable to choose a random card from the deck
var card = deck[Math.floor(Math.random()*deck.length)],
card_remove = card,
position = deck.indexOf(card);
if (~position) deck.splice(position, 1);
// checking that a card is picked and that it is removed from deck
console.warn(card)
console.warn(deck)
Next up I will need to look at storing the card values in the players hand or the dealers hand and checking if the values have gone over 21.
/*Object Oriented Blackjack |
A Game has: Players |
Dealer |
Deck |
A Player / Dealer has: Score |
Cards |
A Score has: Game Logic |
Current Score |
A Deck has: Cards |
*/ |
function Game() { |
this.currentTurnIndex = 0; |
this.deck = new Deck(); |
} |
function Deck() { |
this.cards = []; |
this.cardsDrawn = 0; |
var suits = ['spades', 'diamonds', 'hearts', 'clubs']; |
var names = ['ace', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'jack', 'queen', 'king']; |
for (var suit in suits) { |
for (var name in names) { |
this.cards.push(new Card(names[name], suits[suit])); |
} |
} |
} |
Deck.prototype.getCard = function () { |
if (this.cards.length this.cardsDrawn) { |
return null; |
} //case: check if all cards drawn |
var random = Math.floor(Math.random() * (this.cards.length - this.cardsDrawn)); |
var temp = this.cards[random]; |
//swap chosen card with card last in array |
this.cards[random] = this.cards[this.cards.length - this.cardsDrawn - 1]; |
this.cards[this.cards.length - this.cardsDrawn - 1] = temp; |
this.cardsDrawn++; |
return temp; |
}; |
function Card(name, suit) { |
this.name = name; |
this.suit = suit; |
} |
Card.prototype.image = function () { |
return 'http://www.jonarnaldo.com/sandbox/deck_images/' + name + '_of_' + suit + '.png'; |
}; |
Card.prototype.value = function () { |
if (this.name 'jack' 'queen' 'king') { |
return [10]; |
} else if (this.name 'ace') { |
return [1, 11]; |
} else { |
return parseInt(this.name, 10); |
} |
}; |
function Player() { |
//this.name; |
this.cards = []; |
} |
Player.prototype.addCard = function () { |
this.cards.push(deck.getCard()); |
}; |
Player.prototype.score = function () { |
var score = 0; |
var aces = []; |
for (var i = 0; i < this.cards.length; i++) { |
var value = this.cards[i].value() // value array ex.[10] |
if (value.length 1) { |
score += value[0]; |
} else { |
aces.push(value); |
} |
} |
for (var j = 0; j < aces.length; j++) { |
if (score + aces[j].value[1] <= 21) { |
score + aces[j].value[1]; |
} else { |
score + aces[j].value[0]; |
} |
} |
return score; |
}; |
var deck = new Deck(); |
var player1 = new Player(); |
$('#getCard').click(function () { |
player1.addCard(); |
var cardName = player1.cards[player1.cards.length-1].name; |
var cardSuit = player1.cards[player1.cards.length-1].suit; |
$('#table').append(cardName + cardSuit); |
}); |
commented Jun 13, 2017
My coding bootcamp is making me code a Blackjack Hand Calculator. I have to turn it in soon. I'm failing. JavaScipt is fking me. |